WordPress is a popular Content Management System (CMS), powering over 43% of the web. To improve the security, performance, and scalability of WordPress applications, developers decouple their WordPress content from their web infrastructure.
This post will show how to use headless WordPress with a frontend framework (Next.js) on secure, fast infrastructure (Vercel). The completed example includes:
- Instant page loads by prerendering HTML from your WordPress content
- Flexible data fetching using GraphQL with the WPGraphQL plugin
- Updating content without deploying using Incremental Static Regeneration (ISR)
Headless architecture decouples your frontend and backend into separate, independently scalable pieces of infrastructure. Headless WordPress enables you to keep your existing content workflows in place while evolving your frontend for increased performance.
While your frontend can consume the WordPress REST API to display your content, WordPress plugins (like WPGraphQL) enable you to generate a GraphQL API with zero configuration.
Decoupling your frontend and CMS-backed content provides many benefits:
- Flexible: You can use Next.js, Svelte, or any other frontend framework your team prefers and evolve your frontend separately over time.
- Resilient: If your WordPress server has downtime, your Vercel-hosted frontend will continue to serve your traffic without interruption, showing the last statically generated content from the cache. Once your WordPress server is back online, you can then start displaying new content.
- Easy & Instantaneous Content Updates: In a WordPress dashboard, anyone can keep content up to date. Developers can keep their focus on building applications while others make content changes. Also, with Vercel's Incremental Static Regeneration, changes are propagated globally in less than 500ms.
Let's learn how to use Headless WordPress with your Next.js application.
You’ll need a WordPress server to work with. If you don’t already have one, you can get started locally with a tool like Local.
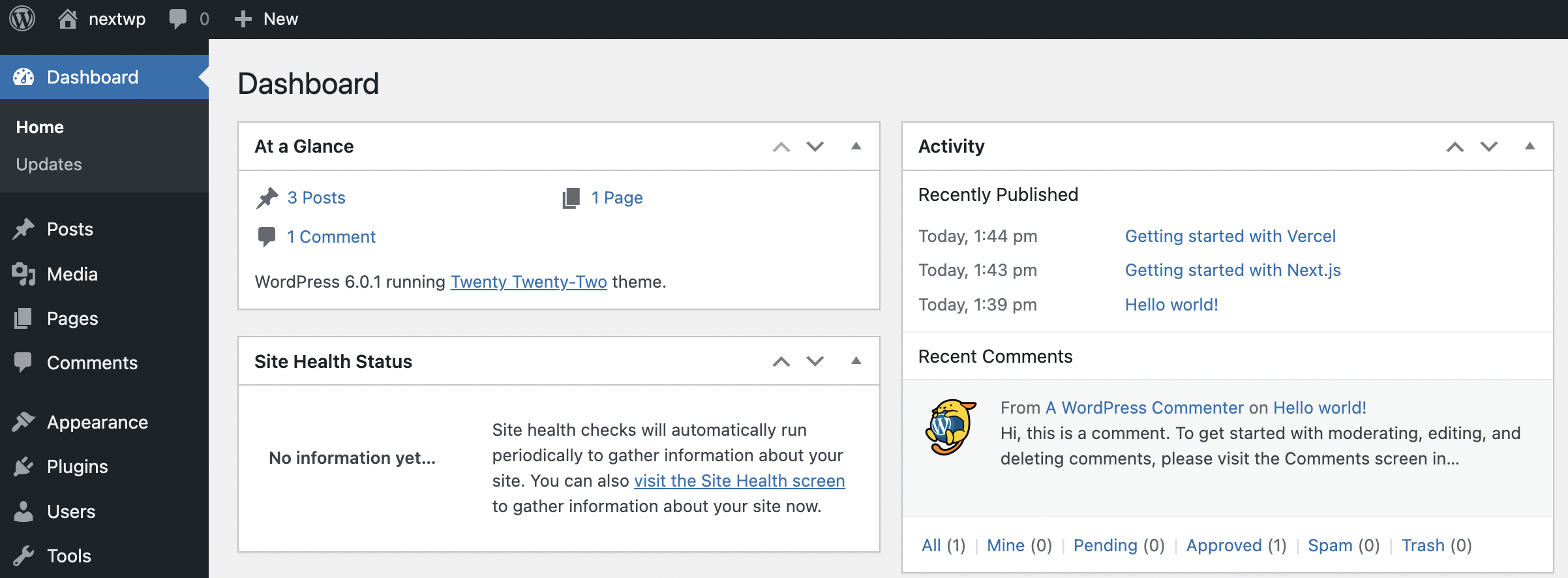
In your WordPress dashboard, go to Plugins, click Add New, and then type GraphQL in the plugin search. Find WPGraphQL, click Install Now, and then Activate the plugin.
This creates a new section for GraphQL in the side navigation. From here, you can access the GraphQL IDE, where you can write and test your queries and configure the plugin Settings.
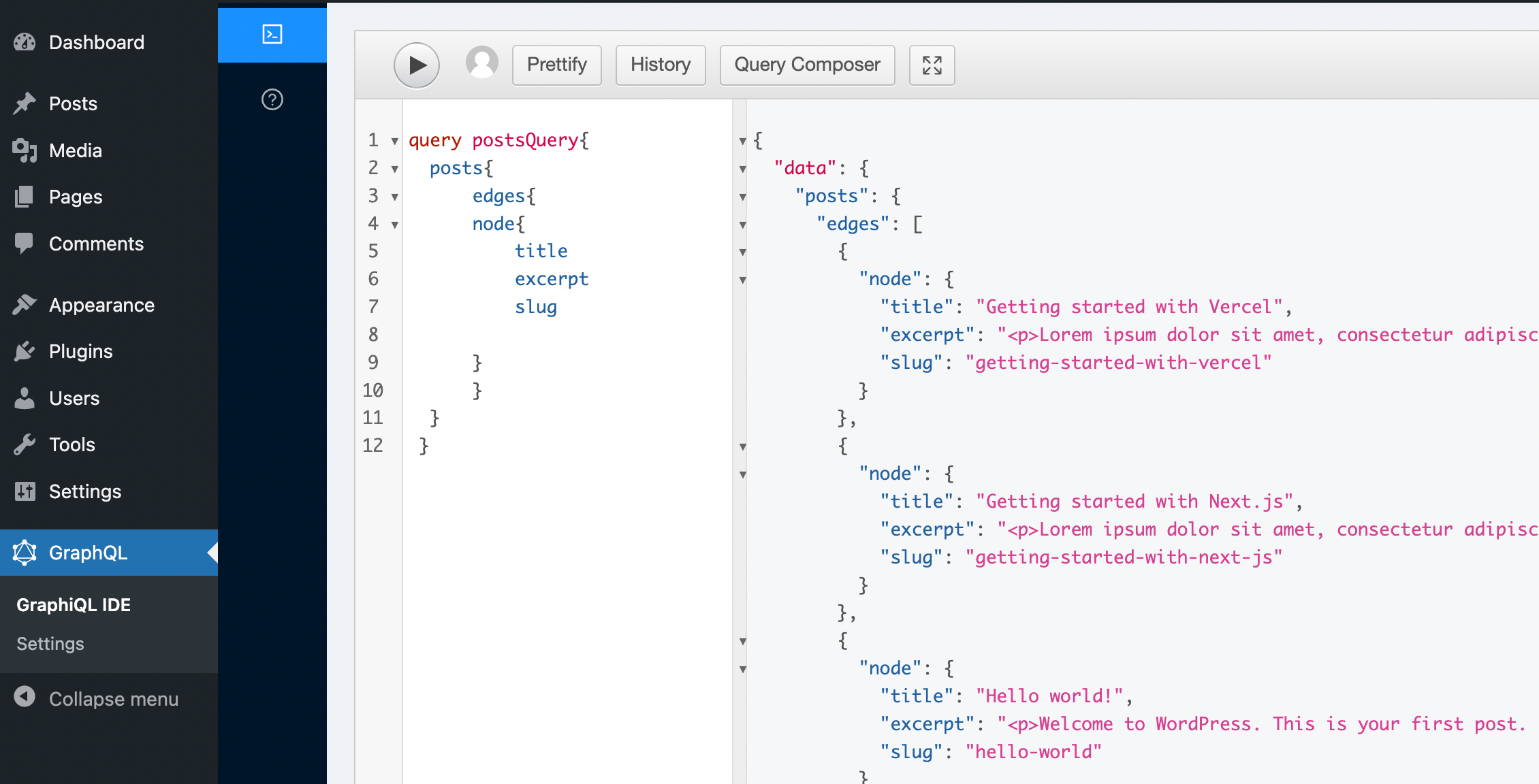
The cms-wordpress
starter example is built using the default Next.js template with some additional blog features.
To get started, run the command for your package manager:
npx create-next-app --example cms-wordpress cms-wordpress-app
In the root directory, copy the .env.local.example
to a new .env.local
file and set the WORDPRESS_API_URL
to your GraphQL API.
# .env.localWORDPRESS_API_URL=
Find the endpoint in the GraphQL Settings section of the WordPress dashboard. WPGraphQL’s default is /graphql
.
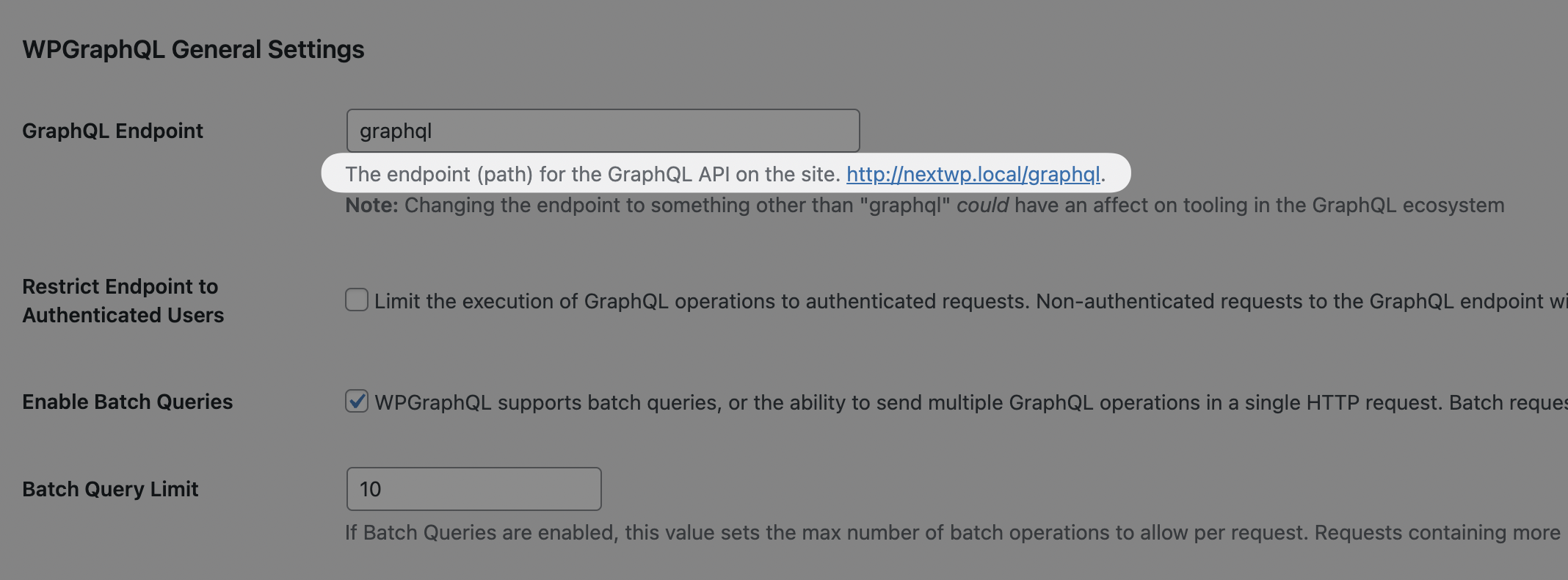
Next, run npm install
to install project dependencies, and npm run dev
to start the local server.
You can now view the local version of your app at http://localhost:3000
. The blog post content on your home page is sourced from your WordPress backend.
Incremental Static Regeneration (ISR) allows you to update your content shown from WordPress without needing to redeploy your website.
To fetch data from WordPress and create statically rendered pages, Next.js uses the getStaticProps()
function. We can use the revalidate
property to define a time interval to check for content updates from WordPress in the background, triggered by an incoming request to your page. To find out more, check out the documentation.
export async function getStaticProps({ preview = false }) { const allPosts = await getAllPostsForHome(preview)
return { props: { allPosts, preview }, revalidate: 10, }}
Using ISR extends static-site generation with an additional revalidate
property that ensures after how many seconds your page gets revalidated. During revalidation, you receive the cached version first and then the updated version (if it exists). This mode of caching is generally known as “stale-while-revalidate.”
The getAllPostsForHome()
function will run server-side at build time, fetching your data from the WordPress database and pre-rendering the content.
// Get the first 20 posts from WordPress, ordered by the dateexport async function getAllPostsFromWordPress(preview) { const data = await fetchAPI(` query AllPosts { posts(first: 20, where: { orderby: { field: DATE, order: DESC } }) { edges { node { title excerpt slug date } } } } `)
return data.posts}
Let’s deploy your Next.js application to Vercel. You can deploy using the cms-wordpress
template in a few minutes from our templates marketplace.
You'll add your WORDPRESS_API_URL
value as an Environment Variable during the deployment to securely connect to your GraphQL API.
Your new headless architecture built on WordPress and Vercel is now more flexible, resilient, and faster. Incremental Static Regeneration will keep your pages in sync with your WordPress content, which Vercel ensures your site is both reliable and fast.